Building a Secure API Gateway with NGINX & OAuth 2.0
Looking for expert solutions in AI, Web Applications, APIs, or blockchain development?
Request a Free ConsultationBuilding a Secure API Gateway with NGINX, OAuth 2.0, Microservices, and an Authenticated Web Interface: A Comprehensive Tutorial
Welcome to this in-depth tutorial! We’ll create a secure API gateway using NGINX, enable HTTPS with Certbot, implement OAuth 2.0 authentication with Node.js and MongoDB (including refresh tokens), build sample Node.js microservices secured with OAuth, and develop a robust, authenticated web interface to monitor and test the system. This setup reflects real-world architectures, teaching you how to secure and manage a microservices-based application.
Introduction: Understanding the Components and Purpose
What is NGINX?
NGINX is a high-performance, open-source web server and reverse proxy. It’s like a traffic controller: it receives requests from clients (e.g., browsers or apps), directs them to the right backend services, and handles tasks like load balancing and rate limiting. Here, NGINX will be our API gateway, ensuring secure and efficient request routing.
What is an API Gateway?
An API gateway is a single entry point for all API requests. Think of it as a mall’s main entrance: instead of visitors finding each store’s back door, they go through one gate that guides them. The gateway:
- Routes requests (e.g.,
/api/v1/products
to a product service). - Enforces security (like requiring OAuth tokens).
- Manages traffic (e.g., preventing overloads).
Without it, clients would need direct service URLs, security would be fragmented, and scaling would be complex. NGINX centralizes these functions.
What Are Microservices?
Microservices are small, independent apps that together form a larger system. Unlike a monolithic app (one big codebase), microservices split tasks into separate services—e.g., managing products or orders. They:
- Run independently.
- Communicate via APIs (usually HTTP).
- Scale separately.
In our e-commerce example:
- Product Service: Lists items.
- Order Service: Processes purchases.
- User Service: Manages profiles.
Each will use OAuth for security, ensuring only authorized requests succeed.
Why Build This?
We’re simulating a professional system to learn:
- How to unify client access with a gateway.
- How to secure services with OAuth 2.0.
- How to monitor and test with an authenticated interface.
This mirrors setups at companies like Amazon or Spotify, where security and scalability are paramount.
Prerequisites
You’ll need:
- Operating System: Ubuntu (or similar Linux).
- Domain Name: A domain (e.g.,
api.example.com
) with DNS set to your server’s IP (uselocalhost
locally, but a domain is ideal for HTTPS). - Tools:
- NGINX:
sudo apt install nginx
- Node.js and npm:
sudo apt install nodejs npm
- MongoDB:
sudo apt install mongodb
- Certbot:
sudo apt install certbot python3-certbot-nginx
- Knowledge: Basic terminal, JavaScript, and HTTP (we’ll explain the rest!).
Step 1: Setting Up the NGINX API Gateway with HTTPS
Why NGINX?
NGINX is fast, lightweight, and built for high-traffic scenarios. It’s perfect for routing requests, balancing loads, and enforcing security—like requiring OAuth tokens—making it an ideal API gateway.
Install and Configure NGINX
- Install NGINX: Update packages:
sudo apt update
Install:
sudo apt install nginx
Start and enable:
sudo systemctl start nginx
sudo systemctl enable nginx
Test: Visit http://localhost
(or your server’s IP) to see NGINX’s welcome page.
- Create a Configuration File: NGINX uses config files to define routing and rules:
sudo nano /etc/nginx/sites-available/api-gateway
Add this initial setup:
upstream backend_service1 {
server 127.0.0.1:8080;
server 127.0.0.1:8081;
}
upstream backend_service2 {
server 127.0.0.1:9090;
server 127.0.0.1:9091;
}
server {
listen 80;
server_name api.example.com;
location /api/v1/service1 {
proxy_pass http://backend_service1;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
}
location /api/v1/service2 {
proxy_pass http://backend_service2;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
}
location /health {
return 200 "API Gateway is healthy\n";
add_header Content-Type text/plain;
}
location / {
return 404 "Not Found\n";
}
}
- Enable and Test: Link it:
sudo ln -s /etc/nginx/sites-available/api-gateway /etc/nginx/sites-enabled/
Check syntax:
sudo nginx -t
Reload:
sudo systemctl reload nginx
Secure with HTTPS Using Certbot
HTTPS encrypts traffic, protecting data from interception. Certbot gets free SSL/TLS certificates from Let’s Encrypt.
- Run Certbot:
sudo certbot --nginx -d api.example.com
- Provide an email.
- Agree to terms.
- Redirect HTTP to HTTPS (option 2).
- Full Config: Here’s the complete setup:
upstream backend_service1 {
server 127.0.0.1:8080;
server 127.0.0.1:8081;
}
upstream backend_service2 {
server 127.0.0.1:9090;
server 127.0.0.1:9091;
}
upstream oauth_service {
server 127.0.0.1:3001;
}
upstream interface {
server 127.0.0.1:3002;
}
limit_req_zone $binary_remote_addr zone=apilimit:10m rate=10r/s;
server {
listen 80;
server_name api.example.com;
return 301 https://$host$request_uri;
}
server {
listen 443 ssl;
server_name api.example.com;
ssl_certificate /etc/letsencrypt/live/api.example.com/fullchain.pem;
ssl_certificate_key /etc/letsencrypt/live/api.example.com/privkey.pem;
include /etc/letsencrypt/options-ssl-nginx.conf;
ssl_dhparam /etc/letsencrypt/ssl-dhparams.pem;
access_log /var/log/nginx/api_access.log;
error_log /var/log/nginx/api_error.log;
location /api/v1/service1 {
auth_request /oauth/validate;
limit_req zone=apilimit burst=20 nodelay;
proxy_pass http://backend_service1;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
location /api/v1/service2 {
auth_request /oauth/validate;
proxy_pass http://backend_service2;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
location /oauth/token {
proxy_pass http://oauth_service;
proxy_set_header Host $host;
}
location /oauth/validate {
internal;
proxy_pass http://oauth_service/validate;
proxy_pass_request_body off;
proxy_set_header Authorization $http_authorization;
}
location /dashboard {
auth_request /oauth/validate;
proxy_pass http://interface;
proxy_set_header Host $host;
}
location /health {
return 200 "API Gateway is healthy\n";
add_header Content-Type text/plain;
}
location / {
return 404 "Not Found\n";
}
}
- OAuth: Now protects all service routes and the dashboard.
- Requires
nginx-auth-request
:sudo apt install libnginx-mod-http-auth-request
.
- Apply:
sudo nginx -t
sudo systemctl reload nginx
Step 2: Building OAuth-Secured Microservices
Why Secure Them?
Without security, anyone could access our services. OAuth ensures only requests with valid tokens succeed, protecting sensitive operations like fetching user data or placing orders.
Create Microservices
- Product Service (Ports 8080, 8081) -
product-service.js
:
const express = require('express');
const axios = require('axios');
const app = express();
const port = process.argv[2] || 8080;
const validateToken = async (req, res, next) => {
const token = req.headers['authorization']?.split(' ')[1];
if (!token) return res.status(401).json({ error: 'No token provided' });
try {
await axios.get('http://localhost:3001/oauth/validate', {
headers: { Authorization: `Bearer ${token}` },
});
next();
} catch (error) {
res.status(401).json({ error: 'Invalid token' });
}
};
app.get('/products', validateToken, (req, res) => {
res.json([
{ id: 1, name: 'Laptop', price: 999 },
{ id: 2, name: 'Phone', price: 499 },
]);
});
app.get('/health', (req, res) => {
res.status(200).json({ status: 'UP', port });
});
app.listen(port, () => console.log(`Product Service on port ${port}`));
- Install:
npm install express axios
.
- Order Service (Port 9090) -
order-service.js
:
const express = require('express');
const axios = require('axios');
const app = express();
const validateToken = async (req, res, next) => {
const token = req.headers['authorization']?.split(' ')[1];
if (!token) return res.status(401).json({ error: 'No token provided' });
try {
await axios.get('http://localhost:3001/oauth/validate', {
headers: { Authorization: `Bearer ${token}` },
});
next();
} catch (error) {
res.status(401).json({ error: 'Invalid token' });
}
};
app.post('/orders', express.json(), validateToken, (req, res) => {
res.json({ orderId: 123, status: 'Placed', date: new Date() });
});
app.get('/health', (req, res) => {
res.status(200).json({ status: 'UP', port: 9090 });
});
app.listen(9090, () => console.log('Order Service on port 9090'));
- User Service (Port 9091) -
user-service.js
:
const express = require('express');
const axios = require('axios');
const app = express();
const validateToken = async (req, res, next) => {
const token = req.headers['authorization']?.split(' ')[1];
if (!token) return res.status(401).json({ error: 'No token provided' });
try {
await axios.get('http://localhost:3001/oauth/validate', {
headers: { Authorization: `Bearer ${token}` },
});
next();
} catch (error) {
res.status(401).json({ error: 'Invalid token' });
}
};
app.get('/users/me', validateToken, (req, res) => {
res.json({ id: 1, name: 'Alice', email: 'alice@example.com' });
});
app.get('/health', (req, res) => {
res.status(200).json({ status: 'UP', port: 9091 });
});
app.listen(9091, () => console.log('User Service on port 9091'));
- Run Them:
node product-service.js 8080 & node product-service.js 8081 & node order-service.js & node user-service.js &
Step 3: Implementing OAuth 2.0 with Node.js and MongoDB
OAuth 2.0 Explained
OAuth 2.0 authorizes access without sharing passwords. It’s used in “Login with…” features. Key concepts:
- Roles: Client (app), Resource Owner (user), Authorization Server (issues tokens), Resource Server (our services).
- Tokens: Access Token (short-lived, e.g., 1 hour) for API calls; Refresh Token (long-lived, e.g., 14 days) to renew access.
- Grants:
- Password Grant: User gives credentials to client (used here for simplicity).
- Refresh Token Grant: Uses refresh token to get new access token.
- Flow: Client gets tokens from
/oauth/token
, uses them in requests, refreshes when needed.
Configure MongoDB
MongoDB stores OAuth data persistently.
- Install:
sudo apt install mongodb
sudo systemctl start mongodb
sudo systemctl enable mongodb
- Set Up Database:
mongo
use oauth_db
exit
- Secure (Optional):
use admin
db.createUser({ user: "admin", pwd: "adminpass", roles: [{ role: "root", db: "admin" }] })
Edit /etc/mongod.conf
:
security:
authorization: "enabled"
Restart:
sudo systemctl restart mongodb
OAuth Server
- Initialize:
mkdir oauth-server
cd oauth-server
npm init -y
npm install express oauth2-server mongoose dotenv bcrypt
- Environment -
.env
:
MONGO_URI=mongodb://localhost:27017/oauth_db
PORT=3001
- Models -
models.js
:
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const ClientSchema = new Schema({
id: { type: String, required: true, unique: true },
secret: { type: String, required: true },
redirectUris: [String],
grants: [String],
});
const UserSchema = new Schema({
username: { type: String, required: true, unique: true },
password: { type: String, required: true },
});
const TokenSchema = new Schema({
accessToken: { type: String, required: true },
accessTokenExpiresAt: { type: Date, required: true },
refreshToken: { type: String },
refreshTokenExpiresAt: { type: Date },
client: { type: Schema.Types.ObjectId, ref: 'Client', required: true },
user: { type: Schema.Types.ObjectId, ref: 'User', required: true },
});
module.exports = {
Client: mongoose.model('Client', ClientSchema),
User: mongoose.model('User', UserSchema),
Token: mongoose.model('Token', TokenSchema),
};
- Server -
oauth.js
:
require('dotenv').config();
const express = require('express');
const OAuth2Server = require('oauth2-server');
const mongoose = require('mongoose');
const bcrypt = require('bcrypt');
const { Client, User, Token } = require('./models');
const app = express();
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
mongoose.connect(process.env.MONGO_URI, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.error('MongoDB error:', err));
const oauth = new OAuth2Server({
model: {
getClient: async (clientId, clientSecret) => {
const client = await Client.findOne({ id: clientId, secret: clientSecret });
return client ? { id: client.id, redirectUris: client.redirectUris, grants: client.grants } : null;
},
getUser: async (username, password) => {
const user = await User.findOne({ username });
if (user && await bcrypt.compare(password, user.password)) return { id: user._id };
return null;
},
saveToken: async (token, client, user) => {
const newToken = new Token({
accessToken: token.accessToken,
accessTokenExpiresAt: token.accessTokenExpiresAt,
refreshToken: token.refreshToken,
refreshTokenExpiresAt: token.refreshTokenExpiresAt,
client: client.id,
user: user.id,
});
await newToken.save();
return {
accessToken: newToken.accessToken,
accessTokenExpiresAt: newToken.accessTokenExpiresAt,
refreshToken: newToken.refreshToken,
refreshTokenExpiresAt: newToken.refreshTokenExpiresAt,
client: { id: client.id },
user: { id: user.id },
};
},
getAccessToken: async (accessToken) => {
const token = await Token.findOne({ accessToken }).populate('client user');
if (!token || token.accessTokenExpiresAt < new Date()) return null;
return {
accessToken: token.accessToken,
accessTokenExpiresAt: token.accessTokenExpiresAt,
client: { id: token.client.id },
user: { id: token.user.id },
};
},
getRefreshToken: async (refreshToken) => {
const token = await Token.findOne({ refreshToken }).populate('client user');
if (!token || (token.refreshTokenExpiresAt && token.refreshTokenExpiresAt < new Date())) return null;
return {
refreshToken: token.refreshToken,
refreshTokenExpiresAt: token.refreshTokenExpiresAt,
client: { id: token.client.id },
user: { id: token.user.id },
};
},
revokeToken: async (token) => {
const result = await Token.deleteOne({ refreshToken: token.refreshToken });
return result.deletedCount > 0;
},
verifyScope: async (token, scope) => true,
},
grants: ['password', 'refresh_token'],
accessTokenLifetime: 3600,
refreshTokenLifetime: 1209600,
});
async function seedData() {
const clientCount = await Client.countDocuments();
if (clientCount === 0) {
await Client.create({
id: 'client1',
secret: 'secret1',
redirectUris: ['http://localhost/callback'],
grants: ['password', 'refresh_token'],
});
const hashedPassword = await bcrypt.hash('test', 10);
await User.create({ username: 'test', password: hashedPassword });
console.log('Seeded initial client and user');
}
}
seedData();
app.post('/oauth/token', (req, res) => {
const request = new oauth.Request(req);
const response = new oauth.Response(res);
oauth.token(request, response)
.then(token => res.json(response.body))
.catch(err => res.status(400).json({ error: err.message }));
});
app.get('/oauth/validate', (req, res) => {
const request = new oauth.Request(req);
const response = new oauth.Response(res);
oauth.authenticate(request, response)
.then(() => res.status(200).end())
.catch(() => res.status(401).end());
});
app.listen(process.env.PORT, () => {
console.log(`OAuth server running on http://localhost:${process.env.PORT}`);
});
- Run:
node oauth.js
Step 4: Building an Authenticated Web Interface
Why Authenticate?
An unprotected interface could let anyone monitor or test your system. Using OAuth ensures only authorized users access it, aligning with our security goals.
Setup
- Initialize:
mkdir gateway-interface
cd gateway-interface
npm init -y
npm install express ejs axios express-session
- Interface Code -
interface.js
:
const express = require('express');
const axios = require('axios');
const session = require('express-session');
const app = express();
app.set('view engine', 'ejs');
app.use(express.urlencoded({ extended: true }));
app.use(express.static('public'));
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: false,
}));
const services = [
{ name: 'Product Service 1', url: 'http://localhost:8080', path: '/api/v1/service1', health: '/health', test: '/products', method: 'GET' },
{ name: 'Product Service 2', url: 'http://localhost:8081', path: '/api/v1/service1', health: '/health', test: '/products', method: 'GET' },
{ name: 'Order Service', url: 'http://localhost:9090', path: '/api/v1/service2', health: '/health', test: '/orders', method: 'POST' },
{ name: 'User Service', url: 'http://localhost:9091', path: '/api/v1/service2', health: '/health', test: '/users/me', method: 'GET' },
];
async function checkHealth(service) {
try {
const response = await axios.get(`${service.url}${service.health}`);
return { status: response.data.status, port: response.data.port };
} catch (error) {
return { status: 'DOWN', error: error.message };
}
}
async function testService(service, token) {
try {
const config = { headers: { Authorization: `Bearer ${token}` } };
const response = service.method === 'POST' ?
await axios.post(`https://api.example.com${service.path}${service.test}`, {}, config) :
await axios.get(`https://api.example.com${service.path}${service.test}`, config);
return { status: 'Success', data: response.data };
} catch (error) {
return { status: 'Failed', error: error.response?.data || error.message };
}
}
app.get('/login', (req, res) => {
res.render('login', { error: null });
});
app.post('/login', async (req, res) => {
const { username, password } = req.body;
try {
const response = await axios.post('https://api.example.com/oauth/token', {
grant_type: 'password',
username,
password,
client_id: 'client1',
client_secret: 'secret1',
});
req.session.token = response.data.access_token;
res.redirect('/dashboard');
} catch (error) {
res.render('login', { error: 'Invalid credentials' });
}
});
app.get('/dashboard', async (req, res) => {
if (!req.session.token) return res.redirect('/login');
const healthChecks = await Promise.all(services.map(checkHealth));
res.render('dashboard', {
gatewayStatus: 'API Gateway is healthy',
rateLimit: '10 req/s',
services: services.map((s, i) => ({ ...s, health: healthChecks[i] })),
testResult: null,
token: req.session.token,
});
});
app.post('/test', async (req, res) => {
if (!req.session.token) return res.redirect('/login');
const { serviceIndex } = req.body;
const service = services[serviceIndex];
const result = await testService(service, req.session.token);
const healthChecks = await Promise.all(services.map(checkHealth));
res.render('dashboard', {
gatewayStatus: 'API Gateway is healthy',
rateLimit: '10 req/s',
services: services.map((s, i) => ({ ...s, health: healthChecks[i] })),
testResult: { service: service.name, ...result },
token: req.session.token,
});
});
app.get('/logout', (req, res) => {
req.session.destroy(() => res.redirect('/login'));
});
app.listen(3002, () => console.log('Interface at http://localhost:3002'));
- Login Template -
views/login.ejs
:
<!DOCTYPE html>
<html>
<head>
<title>Login - API Gateway Dashboard</title>
<link rel="stylesheet" href="/styles.css">
</head>
<body>
<h1>Login to API Gateway Dashboard</h1>
<% if (error) { %>
<p class="status-down"><%= error %></p>
<% } %>
<form method="POST" action="/login">
<label>Username: <input type="text" name="username" required></label><br>
<label>Password: <input type="password" name="password" required></label><br>
<button type="submit">Login</button>
</form>
</body>
</html>
- Dashboard Template -
views/dashboard.ejs
:
<!DOCTYPE html>
<html>
<head>
<title>API Gateway Dashboard</title>
<link rel="stylesheet" href="/styles.css">
</head>
<body>
<h1>API Gateway Dashboard</h1>
<p>Gateway Status: <span class="<%= gatewayStatus.includes('healthy') ? 'status-up' : 'status-down' %>"><%= gatewayStatus %></span></p>
<p>Rate Limit: <%= rateLimit %></p>
<p><a href="/logout">Logout</a></p>
<h2>Service Status</h2>
<table>
<tr>
<th>Service</th>
<th>Health</th>
<th>Port</th>
<th>Test</th>
</tr>
<% services.forEach((service, index) => { %>
<tr>
<td><%= service.name %></td>
<td class="<%= service.health.status === 'UP' ? 'status-up' : 'status-down' %>"><%= service.health.status %></td>
<td><%= service.health.port || 'N/A' %></td>
<td>
<form method="POST" action="/test">
<input type="hidden" name="serviceIndex" value="<%= index %>">
<button type="submit">Test</button>
</form>
</td>
</tr>
<% }); %>
</table>
<% if (testResult) { %>
<h2>Test Result: <%= testResult.service %></h2>
<p>Status: <span class="<%= testResult.status === 'Success' ? 'status-up' : 'status-down' %>"><%= testResult.status %></span></p>
<pre><%= JSON.stringify(testResult.data || testResult.error, null, 2) %></pre>
<% } %>
</body>
</html>
- CSS -
public/styles.css
:
body {
font-family: Arial, sans-serif;
margin: 20px;
}
h1, h2 {
color: #333;
}
table {
border-collapse: collapse;
width: 100%;
margin-top: 20px;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
.status-up {
color: green;
font-weight: bold;
}
.status-down {
color: red;
font-weight: bold;
}
pre {
background-color: #f5f5f5;
padding: 10px;
border-radius: 5px;
}
label {
display: block;
margin: 10px 0;
}
- Run:
node interface.js
Step 5: Testing the System
- Start All:
- NGINX: Already running.
- Microservices:
node product-service.js 8080 & node product-service.js 8081 & node order-service.js & node user-service.js &
- OAuth:
cd oauth-server && node oauth.js &
- Interface:
cd gateway-interface && node interface.js &
- Get a Token:
curl -X POST https://api.example.com/oauth/token \
-d "grant_type=password&username=test&password=test&client_id=client1&client_secret=secret1"
Response:
{
"access_token": "abc123",
"token_type": "Bearer",
"expires_in": 3600,
"refresh_token": "xyz789"
}
- Test Interface:
- Visit
https://api.example.com/dashboard
. - Log in with
test
/test
. - Check health and test services (token is auto-applied).
- Refresh Token:
curl -X POST https://api.example.com/oauth/token \
-d "grant_type=refresh_token&refresh_token=xyz789&client_id=client1&client_secret=secret1"
Conclusion
You’ve learned:
- NGINX: Centralizes traffic and security.
- Microservices: Secured with OAuth for controlled access.
- OAuth 2.0: Protects services and the interface.
- Authenticated Interface: Ensures only authorized monitoring.
For production, add HTTPS to internal services and improve session security. You’ve built a secure, professional system—great job!
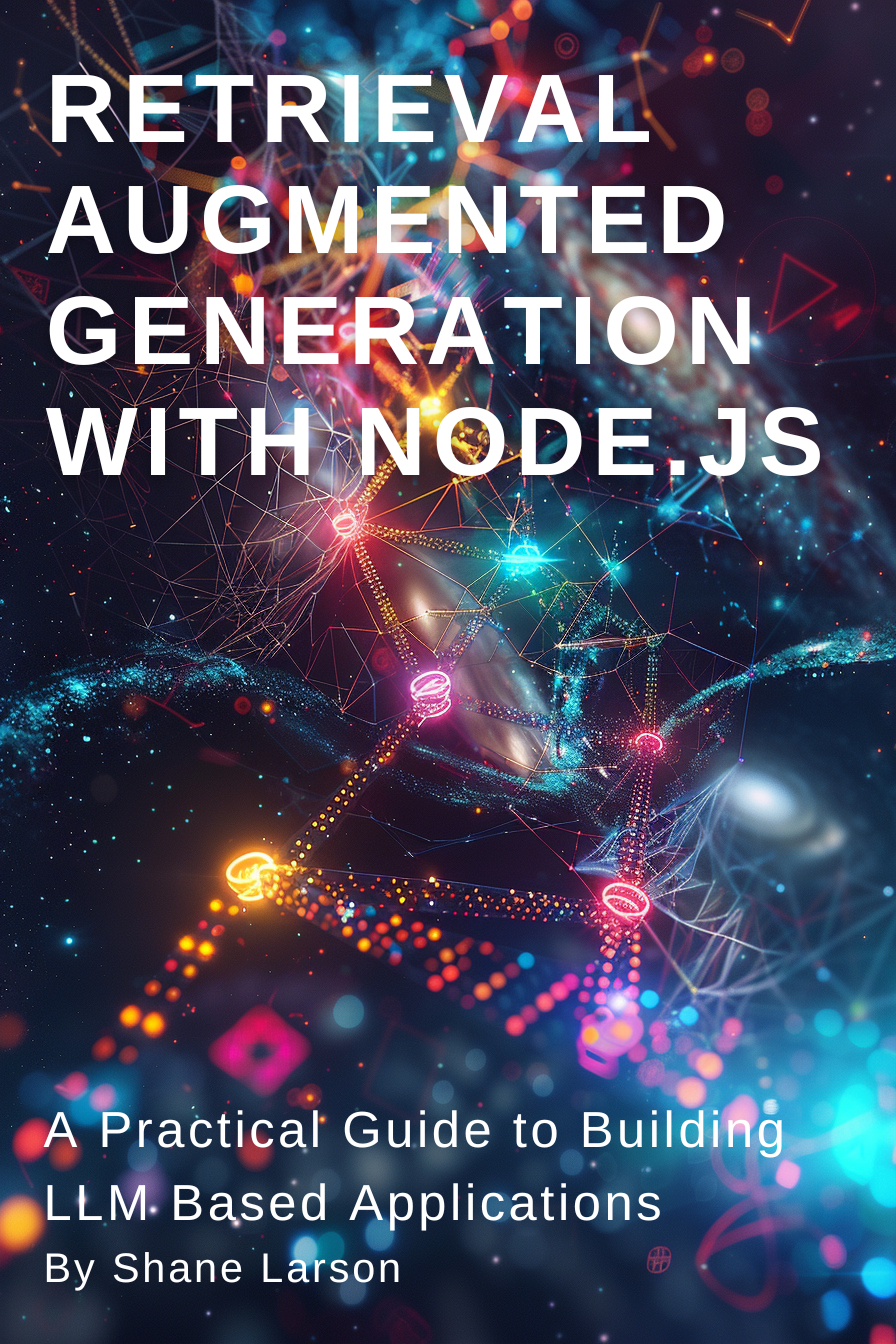
Retrieval Augmented Generation with Node.js: A Practical Guide to Building LLM Based Applications
"Unlock the power of AI-driven applications with RAG techniques in Node.js, from foundational concepts to advanced implementations of Large Language Models."
Get the Kindle Edition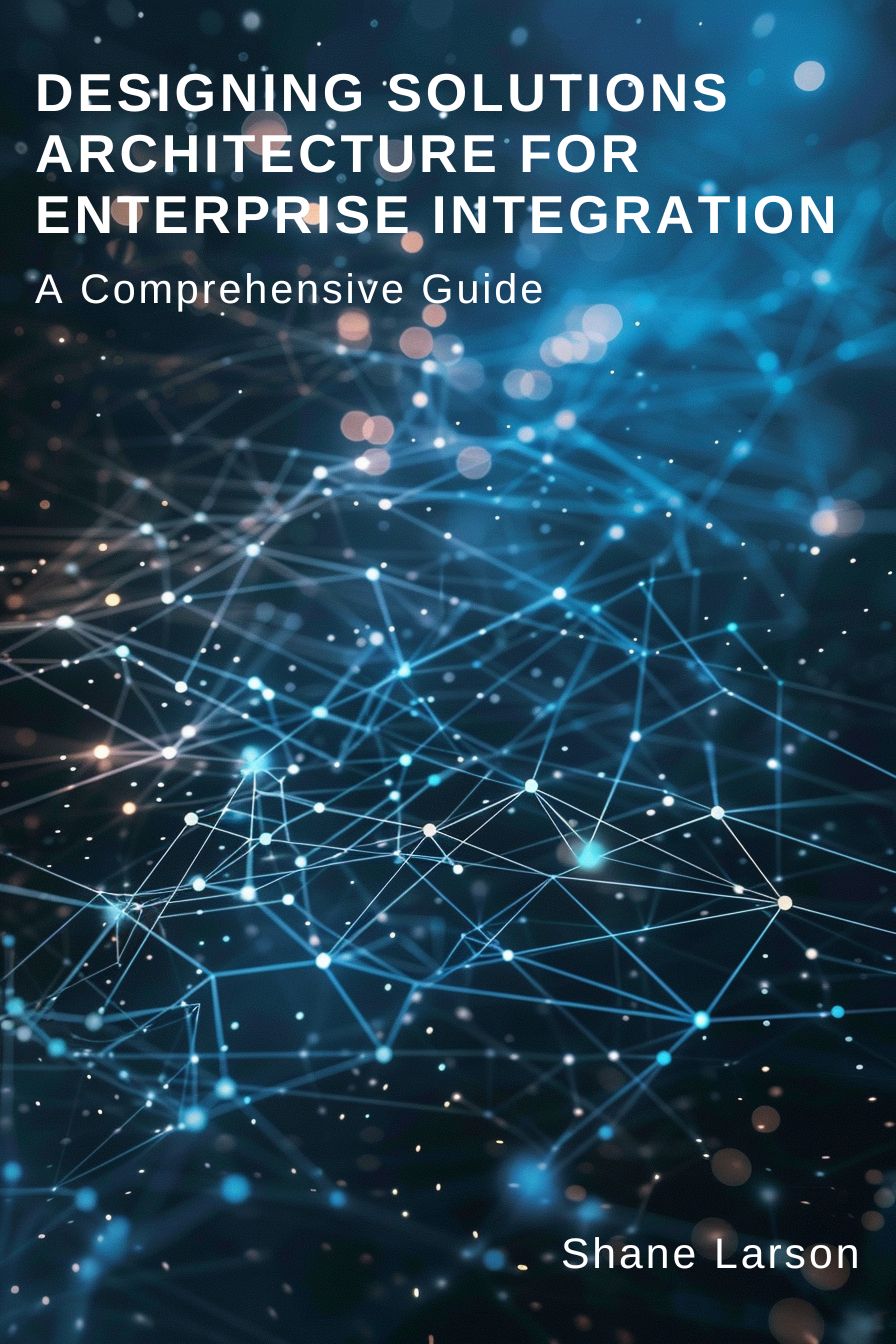
Designing Solutions Architecture for Enterprise Integration: A Comprehensive Guide
"This comprehensive guide dives into enterprise integration complexities, offering actionable insights for scalable, robust solutions. Align strategies with business goals and future-proof your digital infrastructure."
Get the Kindle EditionWe create solutions using APIs and AI to advance financial security in the world. If you need help in your organization, contact us!
Cutting-Edge Software Solutions for a Smarter Tomorrow
Grizzly Peak Software specializes in building AI-driven applications, custom APIs, and advanced chatbot automations. We also provide expert solutions in web3, cryptocurrency, and blockchain development. With years of experience, we deliver impactful innovations for the finance and banking industry.
- AI-Powered Applications
- Chatbot Automation
- Web3 Integrations
- Smart Contract Development
- API Development and Architecture
Ready to bring cutting-edge technology to your business? Let us help you lead the way.
Request a Consultation Now