How to Build and Deploy a Time-Locked Crypto Piggy Bank Using Solidity and Ganache
Looking for expert solutions in AI, Web Applications, APIs, or blockchain development?
Request a Free ConsultationAt Grizzly Peak Software, we specialize in creating blockchain solutions that are both secure and user-friendly. In this tutorial, we’ll guide you through building a Crypto Piggy Bank smart contract using Solidity and deploying it on a local Ganache blockchain. You'll also learn how to interact with the contract through a user-friendly web interface that allows you to deposit funds, set a lock-up period, check your balance, and withdraw funds after the lockup period expires.
The complete code for the Crypto Piggy Bank project is available in our GitHub repository: CryptoPiggyBank GitHub Repository.
Step 1: Set Up Your Development Environment
Before you begin building the contract and interacting with it, you need to install a few essential tools:
1.1 Install Node.js and npm
- Download Node.js from the official website.
- Verify the installation by running the following commands in your terminal:
node -v
npm -v
1.2 Install Ganache
- Download Ganache from the Truffle Suite website and install the desktop version.
- Open Ganache and start a new workspace. Ganache will generate Ethereum accounts with test Ether, which you’ll use to interact with your contract.
1.3 Install Truffle
- Install Truffle globally using npm:
npm install -g truffle
Step 2: Set Up Your Truffle Project
2.1 Initialize a New Truffle Project
- Create a new directory for your project:
mkdir CryptoPiggyBank
cd CryptoPiggyBank
- Initialize the project with Truffle:
truffle init
This will create the basic structure for your Truffle project, including folders for contracts and migrations.
Step 3: Write the Solidity Smart Contract
3.1 Create the Smart Contract
- In the
contracts/
folder, create a new file calledCryptoPiggyBank.sol
. - Add the following Solidity code to implement the time-locked savings functionality:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract CryptoPiggyBank {
mapping(address => uint256) public balances;
mapping(address => uint256) public lockupTime;
// Deposit funds with a specified lock-up time
function deposit(uint256 lockupPeriod) public payable {
require(msg.value > 0, "Deposit must be greater than 0");
balances[msg.sender] += msg.value;
lockupTime[msg.sender] = block.timestamp + lockupPeriod;
}
// Withdraw funds after the lock-up period
function withdraw(uint256 amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
require(block.timestamp >= lockupTime[msg.sender], "Funds are locked");
payable(msg.sender).transfer(amount);
balances[msg.sender] -= amount;
}
// Get balance
function getBalance() public view returns (uint256) {
return balances[msg.sender];
}
// Get lock-up time for the user
function getLockupTime() public view returns (uint256) {
return lockupTime[msg.sender];
}
}
3.2 Compile the Contract
- In your terminal, compile the contract using Truffle:
truffle compile
This will generate the necessary files in the build/
folder.
Step 4: Write the Migration Script
4.1 Create the Migration File
- In the
migrations/
folder, create a file named2_deploy_contracts.js
. - Add the following code to deploy your contract:
const CryptoPiggyBank = artifacts.require("CryptoPiggyBank");
module.exports = function (deployer) {
deployer.deploy(CryptoPiggyBank);
};
Step 5: Configure Ganache
- Open Ganache and start a new workspace. Ganache will provide Ethereum accounts with test Ether.
- In MetaMask, set up a custom network by navigating to Settings > Networks > Add Network:
- Network Name:
Ganache
- New RPC URL:
http://127.0.0.1:7545
- Chain ID:
1337
- Currency Symbol:
ETH
Step 6: Deploy the Contract
- Run the migration to deploy the contract to the local Ganache blockchain:
truffle migrate --reset --network development
- The contract's address will be displayed in the terminal. Note this address for interaction.
Step 7: Interact with the Smart Contract via the Web Interface
Now, let’s set up a simple and intuitive web interface to interact with the deployed contract. This interface will allow users to deposit funds, check their balance, view the lockup period, and withdraw funds.
7.1 Frontend Setup (HTML)
Create an index.html
file in your project’s root directory. This file will allow users to deposit funds, set a lock-up period, check their balance, and withdraw funds.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Crypto Piggy Bank</title>
<link rel="stylesheet" href="styles.css">
<script src="https://cdn.jsdelivr.net/npm/web3/dist/web3.min.js"></script>
</head>
<body>
<div class="container">
<header>
<h1>Crypto Piggy Bank</h1>
<p>Deposit ETH, set a lock-up period, and withdraw after the specified time!</p>
</header>
<section class="deposit-section">
<h2>Make a Deposit</h2>
<div class="input-group">
<label for="depositAmount">Amount to Deposit (ETH):</label>
<input type="number" id="depositAmount" placeholder="Amount in ETH" min="0.1" step="0.1">
</div>
<div class="input-group">
<label for="lockupPeriod">Lock-up Period (seconds):</label>
<input type="number" id="lockupPeriod" value="604800" min="3600">
</div>
<button onclick="depositFunds()">Deposit</button>
</section>
<section class="balance-section">
<h2>Check Balance & Lockup</h2>
<button onclick="checkBalance()">Check Balance</button>
<p id="balance"></p>
<button onclick="checkLockupTime()">Check Lockup Period</button>
<p id="lockupTime"></p>
</section>
<section class="withdraw-section">
<h2>Withdraw Funds</h2>
<div class="input-group">
<label for="withdrawAmount">Amount to Withdraw (ETH):</label>
<input type="number" id="withdrawAmount" placeholder="Amount in ETH">
</div>
<button onclick="withdrawFunds()">Withdraw</button>
</section>
<footer>
<p>Grizzly Peak Software © 2024</p>
</footer>
</div>
<script src="app.js"></script>
</body>
</html>
7.2 JavaScript Setup (app.js)
Create an app.js
file to handle interactions with the Ethereum contract via Web3.js. This file will connect to the local Ganache blockchain and enable you to deposit funds, check balances, view lockup periods, and withdraw funds.
window.addEventListener('load', async () => {
if (typeof window.ethereum !== 'undefined') {
// Connect to the user's Ethereum provider (MetaMask)
const web3 = new Web3(window.ethereum);
await window.ethereum.enable();
const accounts = await web3.eth.getAccounts();
// Address of the deployed contract (use your actual contract address here)
const contractAddress = "<Your_Contract_Address>";
const abi = [ /* ABI from Truffle compile output */ ];
const contract = new web3.eth.Contract(abi, contractAddress);
// Function to deposit funds with lock-up period
window.depositFunds = async () => {
const depositAmount = document.getElementById('depositAmount').value;
const lockupPeriod = document.getElementById('lockupPeriod').value;
if (!depositAmount || depositAmount <= 0) {
alert('Please enter a valid amount to deposit');
return;
}
const depositAmountWei = web3.utils.toWei(depositAmount, 'ether');
try {
await contract.methods.deposit(lockupPeriod).send({
from: accounts[0],
value: depositAmountWei
});
alert(`${depositAmount} ETH
successfully deposited with a lockup period of ${lockupPeriod} seconds`);
} catch (error) {
console.error(error);
alert('Error depositing funds');
}
};
// Function to check the balance of the current account
window.checkBalance = async () => {
try {
const balance = await contract.methods.getBalance().call({ from: accounts[0] });
document.getElementById('balance').innerText = `Balance: ${web3.utils.fromWei(balance, 'ether')} ETH`;
} catch (error) {
console.error(error);
alert('Error checking balance');
}
};
// Function to check the lock-up period for the current account
window.checkLockupTime = async () => {
try {
const lockupTime = await contract.methods.getLockupTime().call({ from: accounts[0] });
document.getElementById('lockupTime').innerText = `Lock-up Time: ${lockupTime}`;
} catch (error) {
console.error(error);
alert('Error checking lockup time');
}
};
// Function to withdraw funds from the contract
window.withdrawFunds = async () => {
const withdrawAmount = document.getElementById('withdrawAmount').value;
if (!withdrawAmount || withdrawAmount <= 0) {
alert('Please enter a valid amount to withdraw');
return;
}
const withdrawAmountWei = web3.utils.toWei(withdrawAmount, 'ether');
try {
await contract.methods.withdraw(withdrawAmountWei).send({ from: accounts[0] });
alert(`${withdrawAmount} ETH successfully withdrawn`);
} catch (error) {
console.error(error);
alert('Error withdrawing funds');
}
};
} else {
alert('Please install MetaMask to use this dApp');
}
});
7.3 Running the Web Interface
To run the index.html
file on a web server, you can use Python or Node.js:
- Using Python:
python3 -m http.server
- Using Node.js:
npm install -g http-server
http-server
Visit http://localhost:8000
(or the port you're using) in your browser to test the application.
Conclusion
By following this tutorial, you’ve created a Crypto Piggy Bank using Solidity and Ganache. The enhanced web interface makes it easier than ever to deposit funds, set lockup periods, check balances, and withdraw funds—all with clear, actionable feedback.
For any custom blockchain solutions or further assistance, feel free to reach out to us at Grizzly Peak Software.
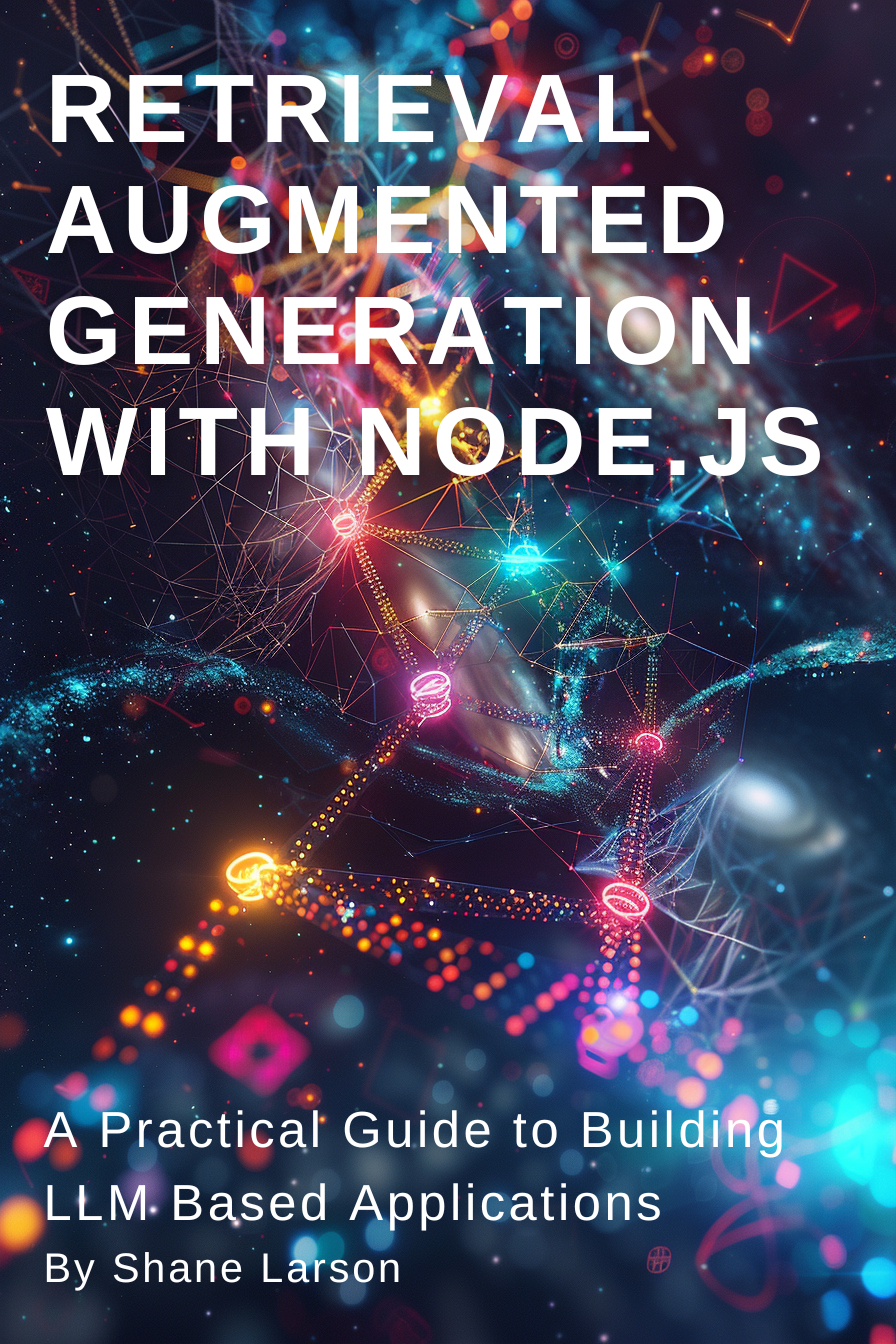
Retrieval Augmented Generation with Node.js: A Practical Guide to Building LLM Based Applications
"Unlock the power of AI-driven applications with RAG techniques in Node.js, from foundational concepts to advanced implementations of Large Language Models."
Get the Kindle Edition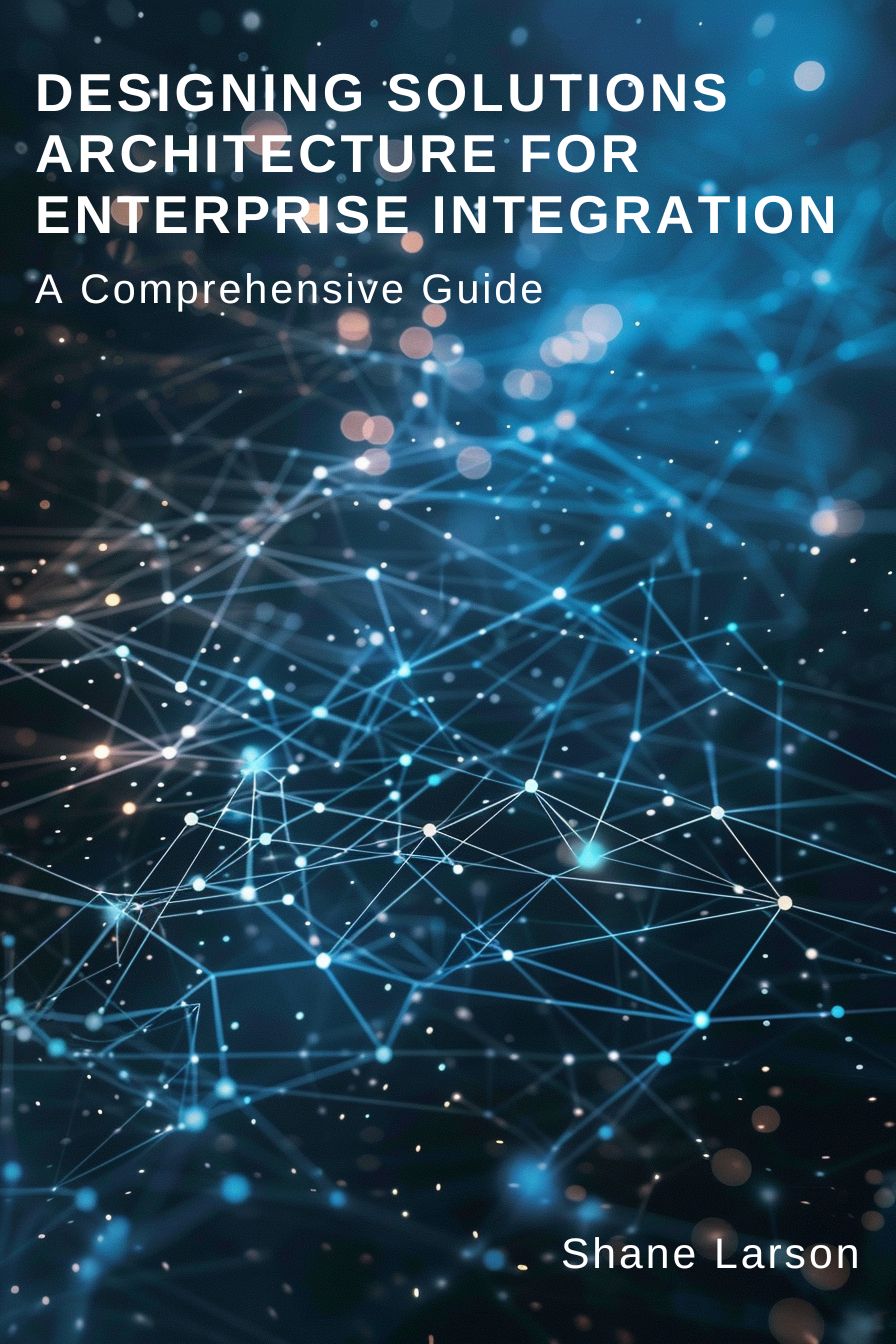
Designing Solutions Architecture for Enterprise Integration: A Comprehensive Guide
"This comprehensive guide dives into enterprise integration complexities, offering actionable insights for scalable, robust solutions. Align strategies with business goals and future-proof your digital infrastructure."
Get the Kindle EditionWe create solutions using APIs and AI to advance financial security in the world. If you need help in your organization, contact us!
Cutting-Edge Software Solutions for a Smarter Tomorrow
Grizzly Peak Software specializes in building AI-driven applications, custom APIs, and advanced chatbot automations. We also provide expert solutions in web3, cryptocurrency, and blockchain development. With years of experience, we deliver impactful innovations for the finance and banking industry.
- AI-Powered Applications
- Chatbot Automation
- Web3 Integrations
- Smart Contract Development
- API Development and Architecture
Ready to bring cutting-edge technology to your business? Let us help you lead the way.
Request a Consultation Now