Creating a Contact Form API with Spam Detection Using ChatGPT
Looking for expert solutions in AI, Web Applications, APIs, or blockchain development?
Request a Free ConsultationCreating a Contact Form API with Spam Detection Using ChatGPT
Introduction
In this tutorial, we will create an open-source API that allows users to submit a contact form from a web page. One of the main challenges of handling contact form submissions is dealing with spam. To address this, we will integrate ChatGPT's API to detect and prevent spam submissions. By the end of this tutorial, you'll have a fully functional API that evaluates contact form submissions for spam before accepting them.
Source Code
You can find the code files for this project here: Contact API Source Code
Setting Up the Project
First, let's set up our project. We'll use Node.js with Express for the server, and we'll need a few other dependencies for handling CORS, parsing request bodies, and making HTTP requests.
Initialize the Project
mkdir contact-form-api cd contact-form-api npm init -y
Install Dependencies
npm install express cors body-parser axios dotenv
Environment Configuration
Next, we need to configure our environment variables. Create a .env
file in the root of your project and add the following variables:
PORT=5000
OPENAI_API_KEY=your_openai_api_key
ALLOWED_ORIGINS=http://localhost:3000,http://example.com
Replace your_openai_api_key
with your actual OpenAI API key, and adjust ALLOWED_ORIGINS
to include the origins you want to allow.
Creating the Express Server
Let's create our server using Express. We'll configure CORS to allow requests from specified origins and set up the body-parser middleware to parse JSON request bodies.
Create a file named app.js
and add the following code:
require('dotenv').config();
const express = require('express');
const cors = require('cors');
const bodyParser = require('body-parser');
const axios = require('axios');
const app = express();
const port = process.env.PORT || 5000;
app.use(bodyParser.json());
const allowedOrigins = process.env.ALLOWED_ORIGINS.split(',');
const corsOptions = {
origin: function (origin, callback) {
if (allowedOrigins.indexOf(origin) !== -1 || !origin) {
callback(null, true);
} else {
callback(new Error('Not allowed by CORS'));
}
},
optionsSuccessStatus: 200
};
app.use(cors(corsOptions));
Implementing the Spam Check
We will use ChatGPT's API to evaluate the content of the contact form submissions for potential spam. Here is the function that performs the spam check:
const checkForSpam = async (content) => {
const prompt = `
Evaluate the following contact form submission for potential spam, risky information, or suspicious content:
"${content}"
Respond with "safe" if it appears to be legitimate, or "spam" if it appears to be spam or risky.
`;
const response = await axios.post(
'https://api.openai.com/v1/chat/completions',
{
model: 'gpt-3.5-turbo',
messages: [{ role: 'system', content: prompt }],
},
{
headers: {
'Authorization': `Bearer ${process.env.OPENAI_API_KEY}`,
'Content-Type': 'application/json',
},
}
);
return response.data.choices[0].message.content.trim().toLowerCase();
};
Handling Form Submissions
Now, let's set up the /api/contact
endpoint to handle form submissions. We will validate the input, check for spam, and respond accordingly.
app.post('/api/contact', async (req, res) => {
const { name, email, subject, message } = req.body;
if (!name || !email || !subject || !message) {
return res.status(400).json({ error: 'All fields are required' });
}
const contactContent = `Name: ${name}, Email: ${email}, Subject: ${subject}, Message: ${message}`;
try {
const result = await checkForSpam(contactContent);
if (result === 'spam') {
return res.status(400).json({ error: 'Your message was identified as spam or risky content.' });
}
res.status(200).json({ message: 'Form submitted successfully' });
} catch (error) {
console.error('Error processing contact form:', error);
res.status(500).json({ error: 'Internal server error' });
}
});
Running and Testing the API
Finally, let's add code to start the server and provide instructions on how to run and test the API.
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Start the Server
npm start
Test the API
You can use Postman or any other API testing tool to test the API. Send a POST request to
http://localhost:5000/api/contact
with a JSON body containingname
,email
,subject
, andmessage
.Example request body:
{ "name": "John Doe", "email": "john.doe@example.com", "subject": "Inquiry", "message": "I would like to know more about your services." }
The server will evaluate the submission and respond with either a success message or an error message if the submission is identified as spam.
Conclusion
In this tutorial, we created a contact form API that uses ChatGPT's API to detect and prevent spam submissions. This approach helps ensure that only legitimate messages are processed, reducing the risk of spam and malicious content. This API can be further enhanced with additional features such as email notifications or saving submissions to a database. The possibilities are endless, and you are encouraged to experiment and build upon this foundation. Happy coding!
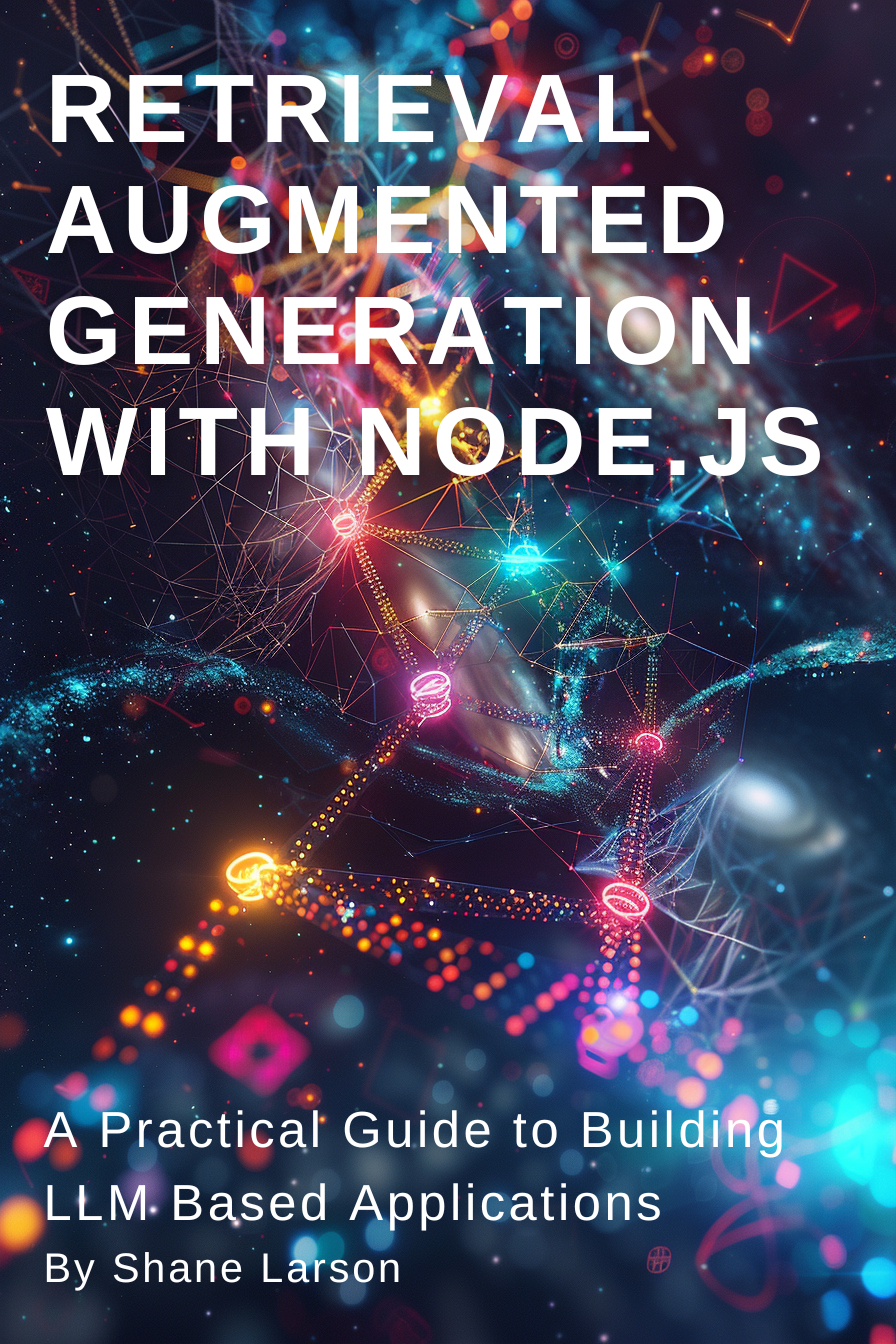
Retrieval Augmented Generation with Node.js: A Practical Guide to Building LLM Based Applications
"Unlock the power of AI-driven applications with RAG techniques in Node.js, from foundational concepts to advanced implementations of Large Language Models."
Get the Kindle Edition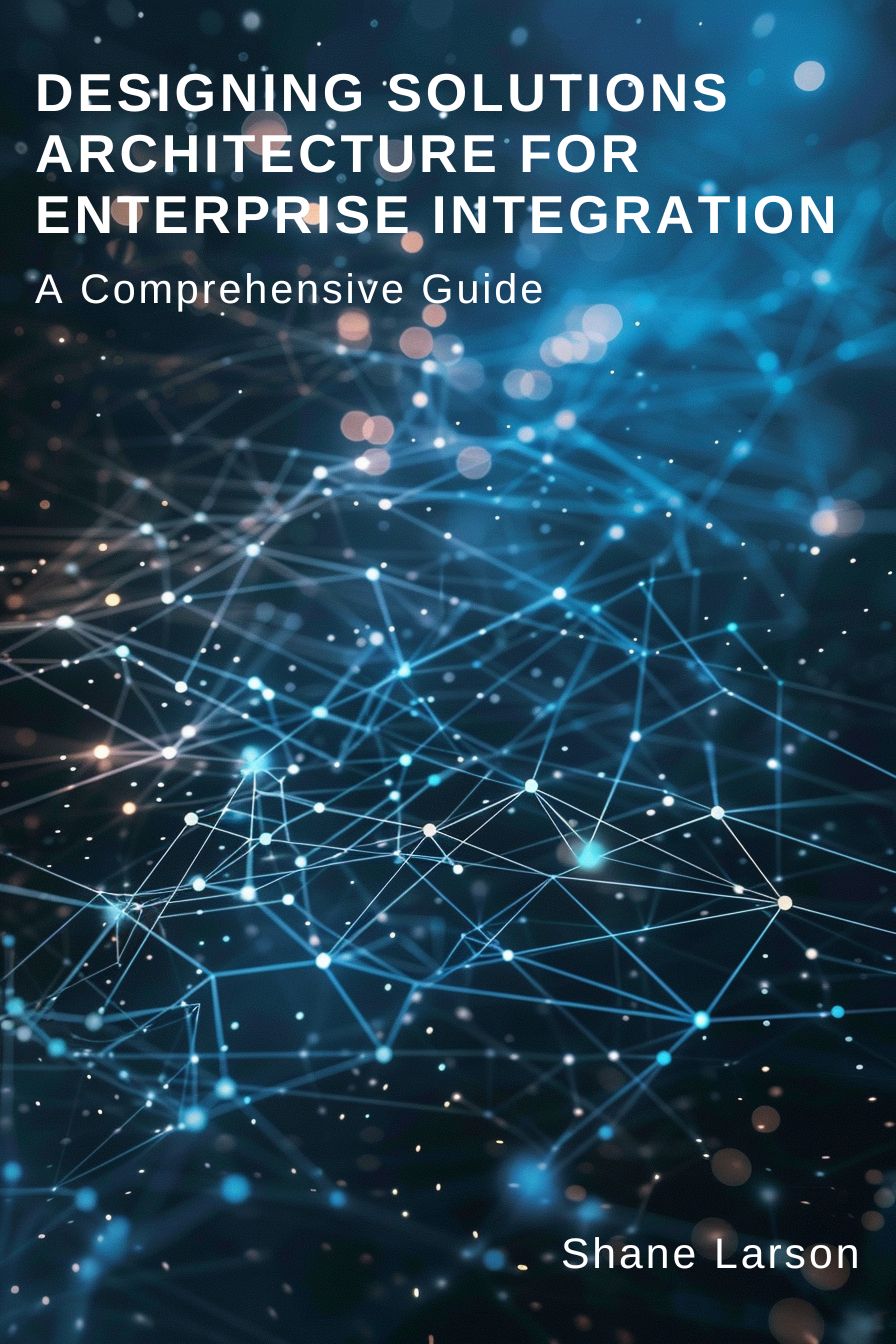
Designing Solutions Architecture for Enterprise Integration: A Comprehensive Guide
"This comprehensive guide dives into enterprise integration complexities, offering actionable insights for scalable, robust solutions. Align strategies with business goals and future-proof your digital infrastructure."
Get the Kindle EditionWe create solutions using APIs and AI to advance financial security in the world. If you need help in your organization, contact us!
Cutting-Edge Software Solutions for a Smarter Tomorrow
Grizzly Peak Software specializes in building AI-driven applications, custom APIs, and advanced chatbot automations. We also provide expert solutions in web3, cryptocurrency, and blockchain development. With years of experience, we deliver impactful innovations for the finance and banking industry.
- AI-Powered Applications
- Chatbot Automation
- Web3 Integrations
- Smart Contract Development
- API Development and Architecture
Ready to bring cutting-edge technology to your business? Let us help you lead the way.
Request a Consultation Now