Understanding the Model Context Protocol (MCP) and Building Your First Memory Server
Looking for expert solutions in AI, Web Applications, APIs, or blockchain development?
Request a Free ConsultationImagine being able to give AI models like Claude the ability to remember things for you, search through those memories, or even connect to your company's database. That's where the Model Context Protocol (MCP) comes in - and it's revolutionizing how we connect AI to the digital world.
Why MCP is Like a USB-C Port for AI
Traditional methods of connecting AI to external systems are like having a drawer full of different chargers for all your devices - messy, inefficient, and frustrating. Before MCP, each time you wanted an LLM to interact with a new API or data source, you needed to:
- Program custom connectors for that specific API
- Embed those connectors directly into the LLM's training or prompt
- Rebuild the entire integration if you switched AI providers
MCP changes all this by creating a universal standard - just like USB-C replaced a tangle of proprietary connectors with one elegant solution.
With MCP, any compatible AI can understand and interact with any MCP server without specific programming for each connection. This standardization creates an ecosystem where:
- AI developers can focus on making better models instead of building hundreds of API connectors
- Tool developers can build MCP servers once and have them work with any AI that supports MCP
- End users get a plug-and-play experience, connecting their favorite AI to their favorite tools
This universal connectivity is what makes MCP such a breakthrough - it will drastically accelerate the interconnectivity of AI agents, allowing them to access specialized tools and data sources without custom integration work.
What is the Model Context Protocol?
At its core, MCP is an open standard that defines the communication rules between AI models (clients) and external capabilities (servers). It creates a structured way for AI systems to discover, access, and interact with data and functionality provided by external sources.
The protocol works by allowing servers to expose capabilities through a standardized interface that any MCP-compatible client can understand. When an AI like Claude connects to an MCP server, it gets a "menu" of what the server offers and how to use each item.
The Three Pillars of MCP
MCP has three main components that together create a powerful toolkit for expanding AI capabilities:
1. Resources
Resources are pieces of data that AI models can access and read. Think of resources as "GET" endpoints in a REST API - they provide information but don't typically perform actions or change state.
Examples of resources include:
- Files or documents
- Database records
- API responses
- Application state
- System information
Resources are identified by URIs (Uniform Resource Identifiers) and can be static or dynamic. For example, a file resource might have a URI like file:///path/to/document.txt
, while a database record might be database://customers/1234
.
Dynamic resources can use templates with parameters, like user://{id}/profile
, allowing the AI to request specific variations of a resource.
2. Tools
Tools are functions that AI models can invoke to perform actions or computations. Unlike resources (which are primarily about reading data), tools can modify state, perform calculations, or trigger external systems.
Examples of tools include:
- Mathematical calculations
- Database queries or updates
- API calls to external services
- File operations (create, update, delete)
- System commands
Each tool has a name, description, and a schema defining its parameters. The AI model can understand these definitions and correctly invoke the tools with appropriate arguments.
Here's where tools really shine: instead of having to program an AI to understand the specific syntax and behavior of hundreds of different APIs, the AI can discover and use tools through a consistent interface. This means new tools can be added to an ecosystem without requiring changes to the AI model itself.
3. Prompts
Prompts are templated interactions designed to help AI models generate more effective responses for specific tasks. They provide a standardized way to guide the AI's output format and content.
Examples of prompts include:
- Code generation templates
- Data analysis workflows
- Customer service response formats
- Creative writing frameworks
Prompts help ensure consistent, high-quality AI outputs for common tasks. They can include placeholders for dynamic content and can be chained together to create complex workflows.
The Memory Server: What We're Building
Now that we understand what MCP is, let's build something practical with it! Our Memory Server will allow Claude (or any MCP-compatible AI) to:
- Store text memories with optional tags
- Search memories by content or tags
- Retrieve all memories
- Delete memories
- Get a summary of used tags
This server saves memories as JSON files on your local machine, making it a simple but powerful example of what MCP can do.
š Here is a link to the github repo for this project: MCP Memory Server
Building Your Memory Server
Our Memory Server is built using TypeScript and the MCP SDK. Let's break down the key components and how they work together:
Core Components
- MemoryManager: The backend that handles storing, retrieving, and managing memories in a JSON file
- MCP Server: The interface that exposes memory functionality through standardized MCP tools
- Transport Layer: The communication channel connecting the server to MCP clients like Claude Desktop
The Memory Manager
The Memory Manager's job is straightforward: maintain a collection of memories and persist them to disk. Here's the data structure we use:
interface MemoryItem {
id: string; // Unique identifier
content: string; // The memory text
tags: string[]; // Optional categorization tags
createdAt: string; // ISO timestamp
}
The manager provides methods to:
- Initialize and load existing memories
- Save memories to disk
- Store new memories
- Search memories by text or tags
- Get all memories
- Delete memories by ID
- Get a summary of all tags used
One of the most important methods is storeMemory
:
async storeMemory(content: string, tags: string[] = []): Promise<MemoryItem> {
// Generate a unique ID combining timestamp and random string
const id = Date.now().toString(36) + Math.random().toString(36).substring(2, 7);
// Create the memory object
const newMemory: MemoryItem = {
id,
content,
tags,
createdAt: new Date().toISOString()
};
// Add to memory collection
this.memories.push(newMemory);
// Persist to disk
await this.saveMemories();
// Return the new memory
return newMemory;
}
This creates a new memory with a unique ID, adds it to our collection, saves everything to disk, and returns the new memory object.
Exposing Memory Functions as MCP Tools
Now comes the interesting part: exposing our memory functionality as MCP tools that Claude or other AI models can discover and use. Here's how we set up the server:
// Create server
const server = new McpServer({
name: "memory-storage",
version: "1.0.0"
});
Then we define our tools. Let's look at each one:
1. Store Memory Tool
server.tool(
"store-memory", // Tool name
"Store a new memory", // Description
{
content: z.string().describe("The memory content to store"), // Parameter schema
tags: z.array(z.string()).optional()
.describe("Optional tags to categorize the memory") // Optional parameter
},
async ({ content, tags = [] }) => { // Execution function
try {
const memory = await memoryManager.storeMemory(content, tags);
return {
content: [
{
type: "text",
text: `Memory stored successfully with ID: ${memory.id}`
}
]
};
} catch (error) {
return {
isError: true,
content: [
{
type: "text",
text: `Failed to store memory: ${(error as Error).message}`
}
]
};
}
}
);
This defines a tool that:
- Is named "store-memory"
- Has a human-readable description
- Accepts a required "content" parameter and an optional "tags" array
- When executed, calls our MemoryManager to store the memory
- Returns a success message or formatted error
2. Search Memories Tool
server.tool(
"search-memories",
"Search for stored memories",
{
searchTerm: z.string().optional().describe("Text to search for in memories"),
tag: z.string().optional().describe("Filter memories by this tag")
},
async ({ searchTerm = "", tag }) => {
try {
const memories = await memoryManager.searchMemories(searchTerm, tag);
if (memories.length === 0) {
return {
content: [
{
type: "text",
text: "No memories found matching your criteria."
}
]
};
}
const formattedMemories = memories.map(memory => `
ID: ${memory.id}
Date: ${new Date(memory.createdAt).toLocaleString()}
Tags: ${memory.tags.length > 0 ? memory.tags.join(", ") : "none"}
Content: ${memory.content}
--------------------------`).join("\n");
return {
content: [
{
type: "text",
text: `Found ${memories.length} memories:\n${formattedMemories}`
}
]
};
} catch (error) {
return {
isError: true,
content: [
{
type: "text",
text: `Failed to search memories: ${(error as Error).message}`
}
]
};
}
}
);
This tool allows searching by text content, tag, or both, and returns formatted results.
The remaining tools follow a similar pattern:
- get-all-memories: Returns all stored memories
- delete-memory: Removes a specific memory by ID
- get-tags-summary: Shows all tags and their usage counts
Setting Up the Transport Layer
Finally, we need to connect our server to the outside world. For command-line use and integration with Claude Desktop, we use the STDIO transport:
// Connect server to stdio transport
const transport = new StdioServerTransport();
await server.connect(transport);
console.error("Memory MCP Server running on stdio");
This allows the server to receive messages via standard input and send responses via standard output - perfect for connecting to Claude Desktop or using with the MCP Inspector.
Setting Up and Running Your Memory Server
Let's get this server up and running!
Prerequisites
- Node.js (version 16 or higher)
- npm (comes with Node.js)
- Claude Desktop (for testing with Claude)
Installation Steps
- Clone the Memory Server repository:
git clone https://github.com/grizzlypeaksoftware/mcp-memory-server.git
cd mcp-memory-server
- Install dependencies:
npm install
- Build the project:
npm run build
- Run the server directly (for testing):
node build/index.js [storage-path]
Where [storage-path]
is an optional directory where memories will be stored (defaults to current directory).
Debugging with MCP Inspector
Before connecting to Claude, it's a good idea to test your server with the MCP Inspector. This tool helps you verify that your server is working correctly and debug any issues.
To use the Inspector with our Memory Server:
npx @modelcontextprotocol/inspector node build/index.js [storage-path]
This will launch a web interface where you can interact with your server:
What You Can Do with the Inspector
The Inspector provides a comprehensive interface for testing all aspects of your MCP server:
- Server Connection Pane: Shows your server's status and allows reconnection
- Tools Tab: Lists all available tools with their schemas and descriptions
- Resources Tab: Shows all resources (if any are defined)
- Prompts Tab: Shows all prompt templates (if any are defined)
- Notifications Pane: Displays server logs and notifications
Testing Your Memory Tools
Let's walk through testing each of our tools:
- Store a memory:
- Go to the "Tools" tab
- Select "store-memory"
- Enter a test memory in the "content" field (e.g., "Remember to buy milk")
- Add some tags in the "tags" field (e.g., ["shopping", "groceries"])
- Click "Execute"
- Verify you get a success message with a memory ID
- Retrieve all memories:
- Select "get-all-memories"
- Click "Execute" with no parameters
- Verify your stored memory appears in the results
- Search memories:
- Select "search-memories"
- Try searching by text (e.g., "milk")
- Try searching by tag (e.g., "shopping")
- Verify the search returns expected results
- Get tags summary:
- Select "get-tags-summary"
- Execute with no parameters
- Verify your tags appear with their usage counts
- Delete a memory:
- Select "delete-memory"
- Enter the ID of a memory you want to delete
- Verify deletion succeeds
- Confirm the memory is gone by running "get-all-memories" again
This testing process helps ensure everything is working before connecting to Claude Desktop.
Connecting to Claude Desktop
Now that your server is working, let's connect it to Claude Desktop so Claude can use your memory tools!
MacOS Setup
- Open Claude Desktop
- Click on the Claude menu in the menu bar and select "Settingsā¦"
- Click on "Developer" in the sidebar, then "Edit Config"
- This will open the configuration file. Add your server like this:
{
"mcpServers": {
"memory": {
"command": "node",
"args": [
"/ABSOLUTE/PATH/TO/build/index.js",
"/ABSOLUTE/PATH/TO/memories-storage"
]
}
}
}
Replace the paths with the actual absolute paths to your server's index.js file and where you want to store memories.
Windows Setup
The process is similar on Windows:
- Open Claude Desktop
- Click on the Claude menu and select "Settingsā¦"
- Click on "Developer" in the sidebar, then "Edit Config"
- Add your server configuration:
{
"mcpServers": {
"memory": {
"command": "node",
"args": [
"C:\\ABSOLUTE\\PATH\\TO\\build\\index.js",
"C:\\ABSOLUTE\\PATH\\TO\\memories-storage"
]
}
}
}
Make sure to use double backslashes (\\
) in your Windows paths.
Important Windows Troubleshooting
If you're having trouble getting Claude to recognize your MCP server on Windows, you might need to completely reset the application:
- Open Task Manager (Ctrl+Shift+Esc)
- Find all Claude-related processes (there may be multiple)
- End all Claude tasks
- Restart Claude Desktop
This often resolves issues with MCP server registration on Windows. The reason is that Claude Desktop runs multiple processes, and sometimes background processes can remain active even when you "close" the application, preventing proper initialization of new servers.
Checking Server Connection
After restarting Claude Desktop, look for the hammer icon in the bottom-right corner of the chat input area. If you see it, your MCP server is properly connected!
Click the hammer to see a list of available tools from your Memory Server:
Viewing Logs
If something isn't working, you can check Claude's logs:
On MacOS:
tail -n 20 -f ~/Library/Logs/Claude/mcp*.log
On Windows:
Get-Content -Path "$env:APPDATA\Claude\logs\mcp*.log" -Tail 20 -Wait
These logs will show you any errors or issues with your MCP server connection.
Using Your Memory Server with Claude
Now you can ask Claude to use your Memory Server! Here are some example prompts to try:
Storing Memories
- "Please remember that my anniversary is on June 15th"
- "Store this important quote: 'The only way to do great work is to love what you do.' - Steve Jobs"
- "Remember my Wi-Fi password is 'SecurePassword123' and tag it as 'home' and 'security'"
- "Save this recipe for chocolate chip cookies: [recipe details]"
Retrieving Memories
- "What memories do you have about my anniversary?"
- "Show me all memories tagged with 'security'"
- "What recipes have you stored for me?"
- "List all the memories you have"
Managing Memories
- "Delete the memory about my Wi-Fi password"
- "What tags have you used for memories?"
- "How many memories do you have about quotes?"
Claude will use the appropriate memory tools based on your requests, making it feel like it has persistent memory across conversations.
Enhancing the Memory Server with Resources and Prompts
Our current Memory Server focuses on tools, but we could enhance it with resources and prompts as well:
Adding Resources
We could expose memories as resources that can be directly read:
// Add a resource for recent memories
server.resource(
"recent-memories",
"memories://recent",
async (uri) => {
const memories = await memoryManager.getAllMemories();
const recentMemories = memories.slice(0, 5); // Get 5 most recent
return {
contents: [{
uri: uri.href,
text: JSON.stringify(recentMemories, null, 2),
mimeType: "application/json"
}]
};
}
);
// Add a resource template for searching by tag
server.resource(
"tag-memories",
new ResourceTemplate("memories://tag/{tagName}", { list: undefined }),
async (uri, { tagName }) => {
const memories = await memoryManager.searchMemories("", tagName);
return {
contents: [{
uri: uri.href,
text: JSON.stringify(memories, null, 2),
mimeType: "application/json"
}]
};
}
);
These resources would allow Claude to directly access memory data without executing tools.
Adding Prompts
We could add prompt templates to help Claude use the memory system more effectively:
server.prompt(
"remember-event",
{
event: z.string().describe("The event to remember"),
date: z.string().describe("When the event occurs"),
tags: z.array(z.string()).optional().describe("Categories for this memory")
},
({ event, date, tags = [] }) => ({
messages: [{
role: "user",
content: {
type: "text",
text: `I need to remember this event: ${event} on ${date}. Please store this with appropriate tags including ${tags.join(", ")}.`
}
}]
})
);
This would provide a consistent way to ask Claude to remember events.
Troubleshooting
Having issues with your Memory Server? Here are some common problems and solutions:
Server Won't Start
- Check that Node.js is installed and at least version 16 (
node --version
) - Verify all dependencies are installed (
npm install
) - Make sure the build succeeded (
npm run build
) - Check for errors in the build output
- Verify file permissions on the storage directory
Claude Desktop Can't Connect
- Verify the paths in your configuration are absolute and correct
- Make sure the build folder contains the compiled JavaScript files
- Check Claude's logs for errors
- Restart Claude completely (especially on Windows)
Permissions Issues
- Make sure the storage directory is writable
- On MacOS, check if you need to grant permissions to Claude Desktop
- On Windows, avoid storing memories in protected system directories
Windows-Specific Issues
- Remember to use double backslashes in paths
- Kill all Claude processes via Task Manager before restarting
- Make sure Node.js is in your PATH environment variable
- If using PowerShell, you might need to adjust execution policies
Beyond Memory: What Else Can MCP Do?
Our Memory Server is just one example of what you can build with MCP. Here are some other exciting possibilities:
Database Connectivity
Connect Claude to your databases to ask questions about your data:
- SQL queries for business analytics
- Document database exploration
- Time-series data visualization
File Operations
Give Claude the ability to read and write files on your system:
- Generate and edit code files
- Create documentation
- Analyze log files
API Integration
Connect Claude to external services:
- Check weather forecasts
- Send messages to team collaboration tools
- Track shipments or deliveries
- Manage calendar events
Custom Tools
Build specialized tools for your specific needs:
- Domain-specific calculations
- Report generation
- Data transformations
- Document summarization
The possibilities are virtually endless, and because MCP is standardized, anything you build can work with any MCP-compatible AI client.
Conclusion: Why MCP Matters for the Future of AI
The Model Context Protocol represents a significant leap forward in how we integrate AI systems with the rest of our digital world. Its importance comes down to several key factors:
Standardization Drives Innovation
By creating a common language for AI systems to interact with tools and data, MCP frees developers from repeatedly solving the same integration problems. This allows more focus on creating novel, valuable capabilities instead of reinventing connectivity.
Security by Design
MCP's architecture keeps sensitive data under your control. Rather than sending your data to AI providers, you're bringing AI capabilities to your data through controlled interfaces. This addresses one of the major concerns with AI adoption in sensitive domains.
Future-Proofing
As AI models continue to evolve rapidly, MCP provides an abstraction layer that helps your tools remain compatible with future models. An investment in building MCP servers today will continue to pay dividends as the AI ecosystem evolves.
Ecosystem Growth
The open nature of MCP encourages a flourishing ecosystem of interoperable tools and capabilities. As more developers build MCP servers and more AI models support the protocol, the entire field benefits from network effects.
As you build your own MCP servers or integrate existing ones, you're contributing to this ecosystem and helping shape the future of how AI interacts with the world.
Resources and Further Reading
To continue your MCP journey, check out these valuable resources:
MCP Documentation and Tools
MCP Server Collections
- Official MCP Servers Repository
- Awesome MCP Servers - A curated list of great MCP servers
Our Memory Server Example
- MCP Memory Server - The full source code for the Memory Server described in this article
Notable MCP Servers to Try
- File System: Access and manipulate files
- Git: Interact with Git repositories
- PostgreSQL: Query PostgreSQL databases
- Brave Search: Perform web searches
- Google Drive: Access Google Drive files
- Slack: Interact with Slack workspaces
By building your own MCP server or using existing ones, you're helping create a more connected, capable AI ecosystem. The Memory Server we've built is just the beginning of what's possible when we standardize how AI connects to the world.
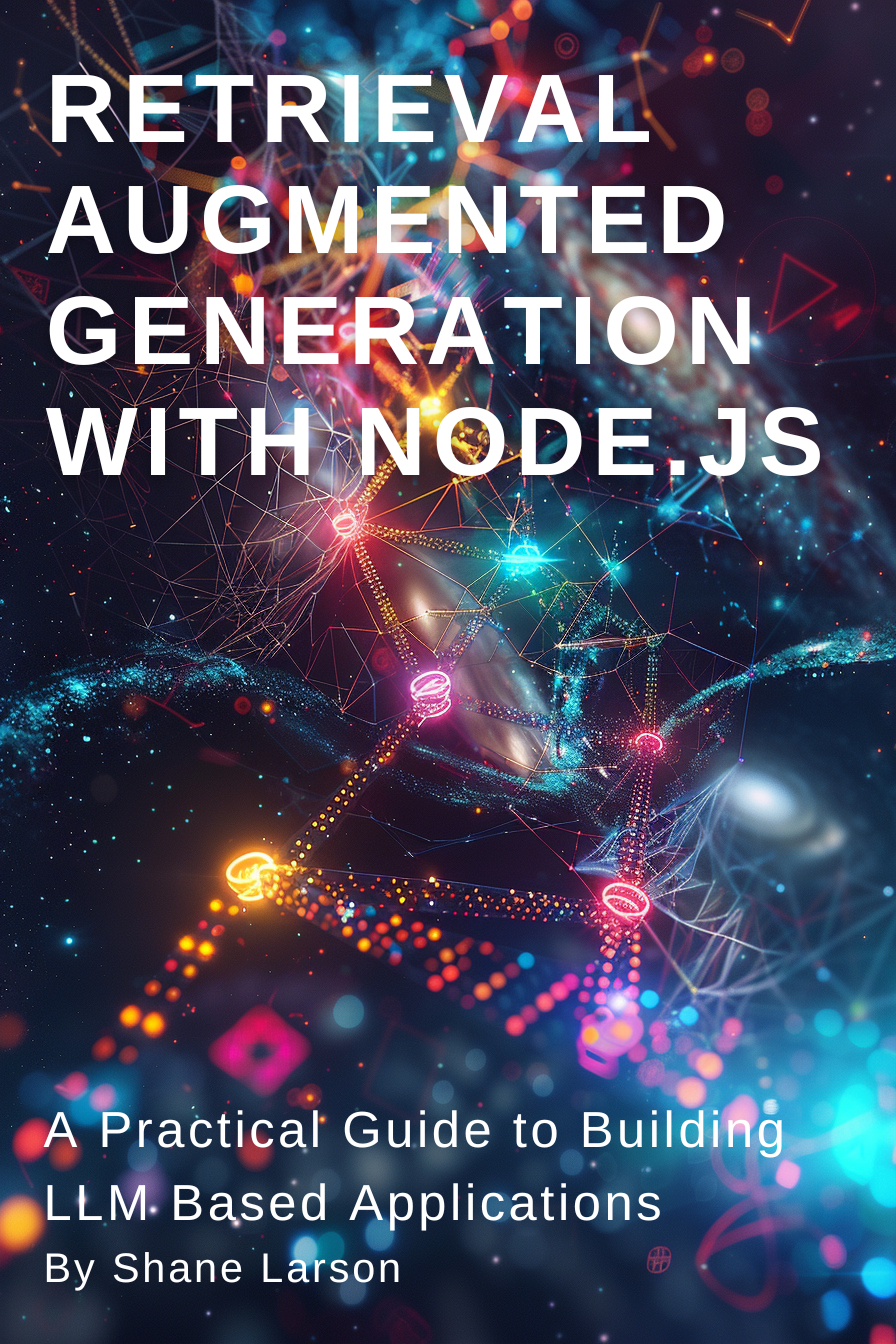
Retrieval Augmented Generation with Node.js: A Practical Guide to Building LLM Based Applications
"Unlock the power of AI-driven applications with RAG techniques in Node.js, from foundational concepts to advanced implementations of Large Language Models."
Get the Kindle Edition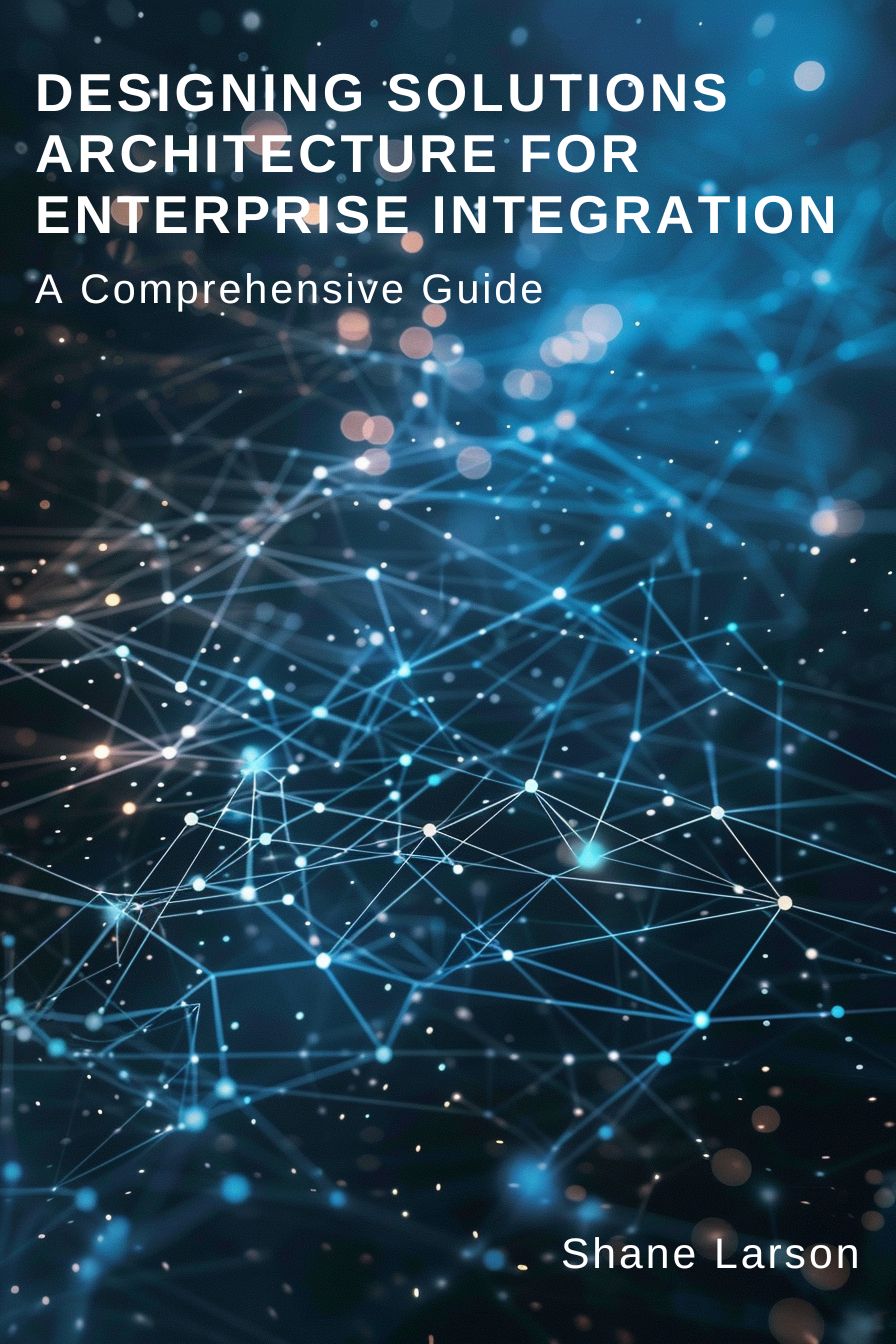
Designing Solutions Architecture for Enterprise Integration: A Comprehensive Guide
"This comprehensive guide dives into enterprise integration complexities, offering actionable insights for scalable, robust solutions. Align strategies with business goals and future-proof your digital infrastructure."
Get the Kindle EditionWe create solutions using APIs and AI to advance financial security in the world. If you need help in your organization, contact us!
Cutting-Edge Software Solutions for a Smarter Tomorrow
Grizzly Peak Software specializes in building AI-driven applications, custom APIs, and advanced chatbot automations. We also provide expert solutions in web3, cryptocurrency, and blockchain development. With years of experience, we deliver impactful innovations for the finance and banking industry.
- AI-Powered Applications
- Chatbot Automation
- Web3 Integrations
- Smart Contract Development
- API Development and Architecture
Ready to bring cutting-edge technology to your business? Let us help you lead the way.
Request a Consultation Now