Creating a Simple QR Code Generator with Node.js
Creating a Simple QR Code Generator with Node.js
In today's digital age, QR codes have become ubiquitous, offering a quick and easy way to share information. As a developer, you might find yourself needing to generate QR codes programmatically. In this tutorial, we'll walk through the process of creating a simple QR code generator using Node.js.
The Code Repository
Before we dive in, you can find the complete code for this project in our GitHub repository:
https://github.com/grizzlypeaksoftware/qrcode_poc
Feel free to clone or fork this repository to follow along or use it as a starting point for your own projects.
Setting Up the Project
To get started, you can either clone the repository mentioned above or set up the project from scratch:
- If cloning the repository:
git clone https://github.com/grizzlypeaksoftware/qrcode_poc.git
cd qrcode_poc
npm install
- If starting from scratch:
mkdir qr-code-generator
cd qr-code-generator
npm init -y
npm install qrcode
Writing the Code
Now, let's create our QR code generator. If you've cloned the repository, you can find this code in the index.js
file. If you're starting from scratch, create a new file named index.js
and add the following code:
const QRCode = require('qrcode');
// URL to encode in the QR code
const url = 'https://www.grizzlypeaksoftware.com';
// Options for QR code generation
const options = {
errorCorrectionLevel: 'H',
type: 'image/png',
quality: 0.92,
margin: 1,
color: {
dark: '#000000',
light: '#FFFFFF'
}
};
// Generate QR code and save as image
QRCode.toFile('qrcode.png', url, options, function (err) {
if (err) throw err;
console.log('QR code saved!');
});
This script does the following:
- We import the
qrcode
package. - We define the URL we want to encode in the QR code.
- We set up options for the QR code generation, including error correction level, image type, quality, margin, and colors.
- We use the
QRCode.toFile()
method to generate the QR code and save it as a PNG file.
Running the Generator
To generate a QR code, simply run:
node index.js
This will create a file named qrcode.png
in your project directory.
Customizing Your QR Codes
You can easily customize your QR codes by modifying the options
object. Here are some things you can adjust:
errorCorrectionLevel
: Set to 'L', 'M', 'Q', or 'H' (low to high correction)type
: Change to 'image/svg+xml' for SVG outputquality
: Adjust between 0 and 1 for PNG qualitymargin
: Set the white space margin (0 to 4)color
: Modify dark and light colors
Conclusion
And there you have it! With just a few lines of code, you've created a flexible QR code generator using Node.js. This simple script can be easily integrated into larger projects or expanded to include features like batch generation or a web interface.
Remember, the qrcode
package offers many more features, including generating QR codes as data URLs or streams. Be sure to check out the official documentation for more advanced usage.
For a complete working example, don't forget to check out our GitHub repository: https://github.com/grizzlypeaksoftware/qrcode_poc
Happy coding!
This post was written by the team at Grizzly Peak Software. For more tutorials and insights on software development, visit our blog at grizzlypeaksoftware.com.
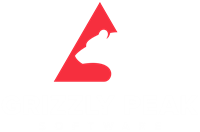
Recent Articles
We create solutions using APIs and AI to advance financial security in the world. If you need help in your organization, contact us!