Unlock Your Web App's Potential with JWT Tokens and Express
Are you tired of managing user logins and passwords for your web application? Do you feel like a medieval gatekeeper with a giant keyring, constantly unlocking doors for users who forget their passwords? Well, have no fear, because JSON Web Tokens (JWT) are here! JWT is a modern solution for authentication that's easier to manage than a hoard of passwords and way less likely to give you carpal tunnel syndrome. With JWT, you can create secure and scalable authentication for your web application, without the hassle of managing user passwords. So grab a cup of coffee (or your preferred beverage), sit back, and let's learn how to use JWT with an Express API.
To use JSON Web Tokens (JWT) with an Express API, you can follow these general steps:
Install the necessary dependencies:
We will use the jsobwebtoken NPM package to help with the JWT.
npm install express jsonwebtoken
Create an endpoint for user authentication.
This endpoint should handle user credentials (e.g., username and password) and generate a JWT token upon successful authentication. Here's an example implementation:
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
// Secret key for JWT
const secretKey = 'your-secret-key';
// Authentication endpoint
app.post('/login', (req, res) => {
// Verify user credentials and generate JWT
const { username, password } = req.body;
if (username === 'user' && password === 'password') {
const token = jwt.sign({ username }, secretKey);
res.json({ token });
} else {
res.status(401).json({ message: 'Invalid credentials' });
}
});
Create Middleware Function
Create a middleware function to verify the JWT on protected endpoints. This middleware function should check for the presence and validity of the JWT token in the request header. Here's an example implementation:
// Middleware to verify JWT
const verifyToken = (req, res, next) => {
const token = req.headers.authorization;
if (!token) {
return res.status(401).json({ message: 'Missing token' });
}
try {
const decoded = jwt.verify(token, secretKey);
req.user = decoded;
next();
} catch (err) {
res.status(401).json({ message: 'Invalid token' });
}
};
Use VerifyToken to Protect Endpoints
Use the verifyToken middleware to protect the API endpoints that require authentication. Here's an example implementation:
// Protected endpoint
app.get('/api/data', verifyToken, (req, res) => {
// Return protected data
res.json({ message: 'Protected data', user: req.user });
});
With these steps, you should be able to use JWT authentication with your Express API. Note that this is just a basic implementation and you may need to customize it depending on your specific requirements.
In summary, JWT tokens provide a way to authenticate users in an Express API by generating and verifying tokens. These tokens are generated using a secret key and can be used to protect routes that require authentication. The jsonwebtoken package provides the necessary functions to generate and verify JWT tokens. By following these steps, you can create a secure and reliable authentication system for your Express API.
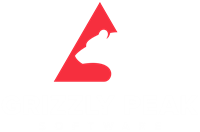
Recent Articles
We create solutions using APIs and AI to advance financial security in the world. If you need help in your organization, contact us!